How To Perform Merge Sort | Sorting Algorithm
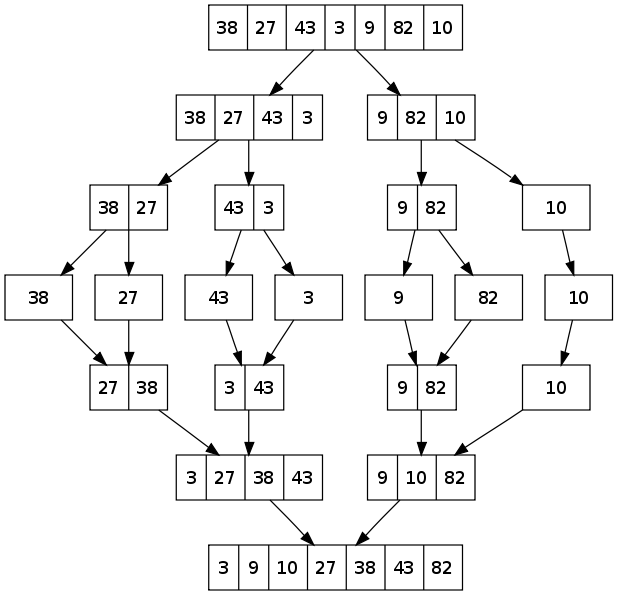
In computer science , merge sort is an efficient, general-purpose, comparison-based sorting algorithm .It is a divide and conquer algorithm that was invented by John von Neumann in 1945. Algorithm Conceptually, a merge sort works as follows: Divide the unsorted list into n sublists, each containing 1 element (a list of 1 element is considered sorted). Repeatedly merge sublists to produce new sorted sublists until there is only 1 sublist remaining. This will be the sorted list. The following diagram shows the complete merge sort process. Source Code: Download the code: Merge.c #include<stdio.h> #include<stdlib.h> void mergesort(int a[],int low,int high) { int mid=(low+high)/2; if(low<high) { mergesort(a,low,mid); mergesort(a,mid+1,high); ...